This is a basic Leafletjs html demo file that we will be building on in subsequent tutorials.
This basic map displays an OpenStreetMap base layer.
<!DOCTYPE html> <html lang="en"> <head> <base target="_top"> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>Basic Leaflet Map</title> <!-- Leafletjs and CSS --> <script src="https://unpkg.com/leaflet@1.9.4/dist/leaflet.js" integrity="sha256-20nQCchB9co0qIjJZRGuk2/Z9VM+kNiyxNV1lvTlZBo=" crossorigin=""></script> <link rel="stylesheet" href="https://unpkg.com/leaflet@1.9.4/dist/leaflet.css" integrity="sha256-p4NxAoJBhIIN+hmNHrzRCf9tD/miZyoHS5obTRR9BMY=" crossorigin=""/> <!-- Inline CSS for map --> <style> html, body { height: 100%; margin: 0; } .leaflet-container { height: 80%; width: 80%; max-width: 100%; max-height: 100%; } </style> </head> <body> <div id="map"></div> <script> // Add OpenStreetMap basemap var map = L.map('map').setView([37.0902, -95.7129], 4); var osm = L.tileLayer('https://tile.openstreetmap.org/{z}/{x}/{y}.png', { maxZoom: 19, attribution: '© <a href="http://www.openstreetmap.org/copyright">OpenStreetMap</a>' }).addTo(map); </script> </body> </html>
This file uses the leafletjs javascript and css files via a CDN url.
<!-- Leafletjs and CSS --> <script src="https://unpkg.com/leaflet@1.9.4/dist/leaflet.js" integrity="sha256-20nQCchB9co0qIjJZRGuk2/Z9VM+kNiyxNV1lvTlZBo=" crossorigin=""></script> <link rel="stylesheet" href="https://unpkg.com/leaflet@1.9.4/dist/leaflet.css" integrity="sha256-p4NxAoJBhIIN+hmNHrzRCf9tD/miZyoHS5obTRR9BMY=" crossorigin=""/>
You can also download the files and serve them locally.
The map div is used to display the generated html content:
<div id="map"></div>
We then initialize the map and set the default view:
var map = L.map('map').setView([37.0902, -95.7129], 4);
Finally, we add an OpenStreetMap layer to serve as our basemap:
var osm = L.tileLayer('https://tile.openstreetmap.org/{z}/{x}/{y}.png', { maxZoom: 19, attribution: '© <a href="http://www.openstreetmap.org/copyright">OpenStreetMap</a>' }).addTo(map);
If we open the file in a browser, we see it displayed as below:
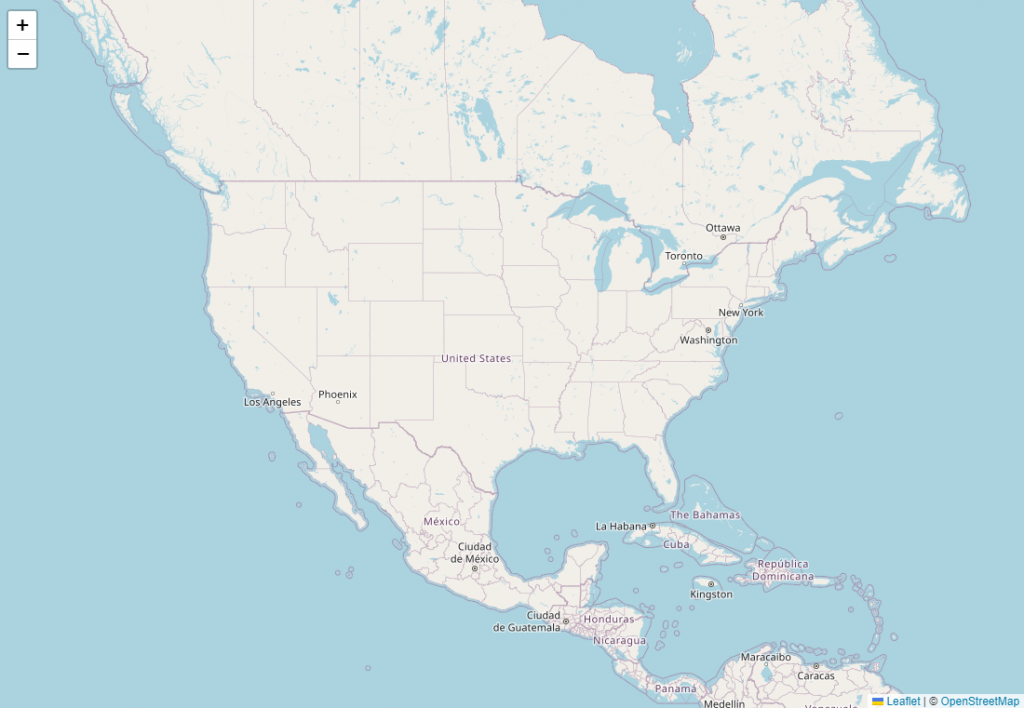
In subsequent tutorials, we will be adding data, layers, controls, styling, and plugins.