OpenLayers Tutorial Part 1: Basic Map
Basic Base Map
OpenLayers is an open-source JavaScript library for mobile-friendly interactive maps. This documentation will help you to create your first map with Leaflet and you will learn some basic mapping principles. Basic usage:
Lets begin with the most basic HTML page:
<html>
<head>
</head>
<body>
</body>
</html>
Include these OpenLayers CSS and Javascript files in head tag:
<script src="https://cdn.rawgit.com/openlayers/openlayers.github.io/master/en/v5.3.0/build/ol.js"></script>
<link rel="stylesheet" href="https://cdn.rawgit.com/openlayers/openlayers.github.io/master/en/v5.3.0/css/ol.css">
Above, we are using a CDN for convenience. For your production maps, use local files as shown in our demo as below:
Include these OpenLayers CSS and Javascript files in head tag:
<link rel="stylesheet" href="OpenLayers/ol.css" type="text/css">
<script src="OpenLayers/ol.js"></script>
Add the map div on the page where you want to display the map and set the height/width for this div
<div id="map"></div>
Use the following minimal JavaScript code inside the <script> tag to initialize the Map.
<script>
var layers = [
new ol.layer.Tile({
source: new ol.source.OSM()
})
];
var map = new ol.Map({
layers: layers,
target: 'map',
view: new ol.View({
center: [-10997148, 4569099],
zoom: 4
})
});
</script>
Putting It All Together
<html>
<head>
<title>OpenLayers Demo</title>
<script src="https://cdn.rawgit.com/openlayers/openlayers.github.io/master/en/v5.3.0/build/ol.js"></script>
<link rel="stylesheet" href="https://cdn.rawgit.com/openlayers/openlayers.github.io/master/en/v5.3.0/css/ol.css">
</head>
<body>
<div id="map" class="map"></div>
<script>
var layers = [
new ol.layer.Tile({
source: new ol.source.OSM()
})
];
var map = new ol.Map({
layers: layers,
target: 'map',
view: new ol.View({
center: [-10997148, 4569099],
zoom: 4
})
});
</script>
</body>
</html>
The resulting HTML document should now look as below. Note that the Zoom function appears in the top lwft corner:
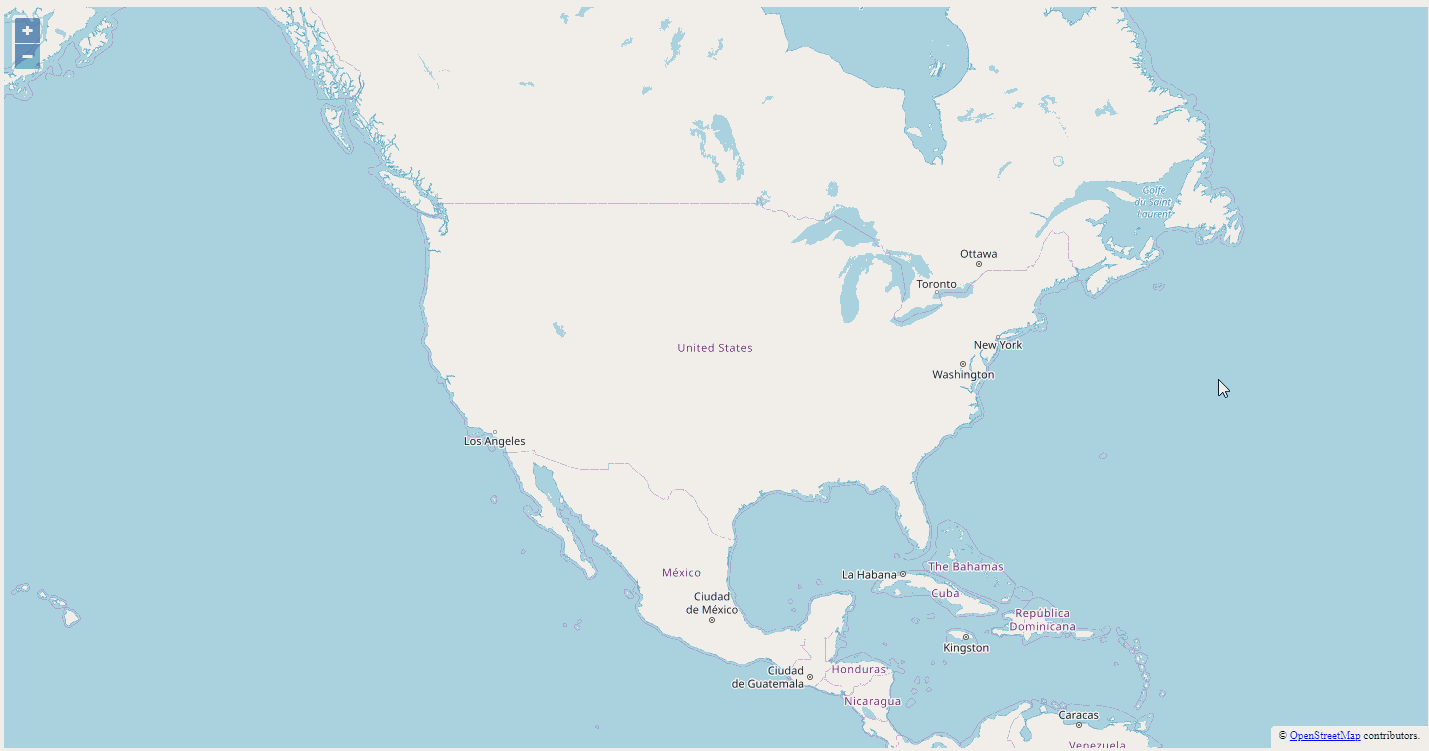
Up Next: OpenLayers Tutorial Part 1